
Every web developer cannot deny the significance of Ajax (Asynchronous JavaScript and XML) in the Rich Internet Applications field (RIA). Thus, I have chosen to begin with how jQuery makes Ajax cleaner and simple to follow.
This is the first article teaching advanced jQuery with a very simple method.
Talking about the XHR instance
First what is XHR?
XHR or XMLHttpRequest is a JavaScript object which helps you to send HTTP requests to a web server and load a response data from this same server.
XHR is your hand to use Ajax in your applications.
The problem with XHR is that different browsers implement it in different ways!
The worst thing is that the XHR is not defined (or not recognized) in some browsers (like IE5 and 6 which are using ActiveXObject instead).
What to do then?
Use the object detection technique which consists to test the browser’s capabilities and not what is the current browser or version used.
And as we want to create an instance of XHR (to play with Ajax), here is a conform manner for all browsers:

Now you can be sure that you have all means to set up (or off) a request to the server thanks to this XHR instance.
But wait HOW?
First we are going to use a property of the XHR instance called: onreadystatechange.
onreadystatechange property (of XHR instance) stores the function that will process the response from the web server (to be called automatically).
And here is the way we will use it for:

What does that mean?
- readyState property is about to show the current state of the request (for more details about the associated numeric codes see this);
- A complete request does not mean of course that it was successful. So this is why we have to check the status property of the XHR instance to know if whether this request was successful or not (Example; 404 for not found and for more details: Check out here).
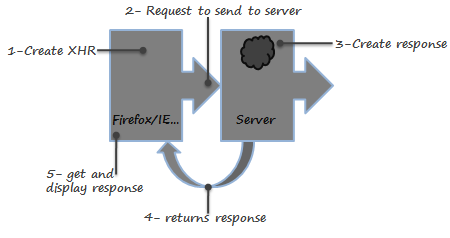
Let’s get into how to deal with the response from a fresh completed request.
Note that the response format can be a kind of plain text, a JavaScript object, an HTML fragment, or a sort of JavaScript Object Notation (JSON) format.
The body content returned in the response can be found in the XHR instance property: responseText.
What is left?
Only two following lines:
xhr.open('GET','/server/application/url');
xhr.send(null);
- The open method defines the HTTP method (GET or POST) and the URL to be used. So we say that it establishes the connection to the server;
- The send method initiates the request. Here we say that it sends the ready request to the server.
xhr.send('x=16&y=17');
To sum up, here is roughly a code snippet to perform properly your Ajax request. ourAjaxDiv is the id of the div in which we want to put our response:
var xhr;
if (window.XMLHttpRequest) {
xhr = new XMLHttpRequest();
}
else if (window.ActiveXObject) {
xhr = new ActiveXObject("Microsoft.XMLHTTP");
}
else {
throw new Error("This browser does not support XHR!”);
}
xhr.onreadystatechange = function() {
if (xhr.readyState == 4) {
if (xhr.status >= 200 && xhr.status < 300) {
//success
document.getElementById('ourAjaxDiv').innerHTML = xhr.responseText;
}
else {
//failure
}
}
}
xhr.open('GET','/server/application/url');
xhr.send(null);
All these code lines above should be compressed in only one line thanks to jQuery:
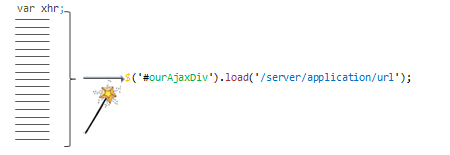
This was the equivalent code in jQuery ! How do you find it?
There is another thing more important than this equivalent code. It is merely the fact that jQuery handles for us quite a few issues that we can have when we get the response body.
For more details about this function, please check out the great documentation of jQuery official site: Link.
This was a first glimpse about the strength of jQuery about how it handles the communication with the server with a clean and easy JavaScript code.
The next articles related to this topic will talk more closely about Ajax handling by jQuery. We will see with examples how we can use some functions like $.getJSON, $.get, $.post, $.ajax and others.
Without your comments and suggestions, this should not succeed. So please don't hesitate to ask!

- Promote our sponsors;
- Add any kind of comment or critic;
- Ask me directly by email if you prefer.






