I wanted to show in this article how possible to create a nice star rater using CSS and jQuery. Every one of us knows how sometimes it is important to embed a star rating feature pretty near an article or a video to allow all visitors to size up this article or video.
In order to shape our star rater, let’s get first as usual to structure.

Our star rater will be a sort of list of links:
HTML
<div id ="stars-container">
<h1>Star Rater with CSS/jQuery</h1>
<ul class='star-rate'>
<li><a href='#' title='' class="star-one">mmmm</a></li>
<li><a href='#' title='' class="star-two">try again</a></li>
<li><a href='#' title='' class="star-three">mmm not bad</a></li>
<li><a href='#' title='' class="star-four">this is cool ya!</a></li>
<li><a href='#' title='' class="star-five">very good</a></li>
</ul>
</div>
<div id ="remark"></div>
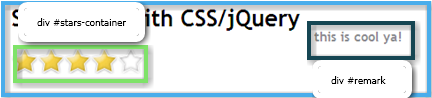
Every anchor item element has a defined class as well as a content which describes what should be displayed when we will hover over a star. For example, if we hover over the second star, the message “try again” will be displayed in the same HTML page.
You could use Ajax to attach different actions to each link.
For example: Store the mark in database that the user has chosen. However, I will not cover that here.
CSS
The ul element CSS class is star-rate:
.star-rate{Now for the anchor items within the list items, we will define the CSS like so:
/* The list contains 5 stars. Each one is 30px, so 5 x 30px = 150px wide */
width: 150px;
/* Each star has 30px height. The list of stars is horizontal list, so its height will be the same one as the star’s one*/
height: 30px;
margin: 0px;
padding:0px;
/* Snuff out the default list image bullets */
list-style:none;
/* We will use later the absolute positioning. So let’s use relatively-absolute positioning */
position: relative;
/* The list will have five stars thanks to repeat-x */
background: url(stars.gif) top left repeat-x;
}
.star-rate li{
float: left;
}
CSS
.star-rate li a{Let’s explain what was not explained above. The specific link that we hover gets pushed all the way to the bottom of the z-index stack (z-index value of “.star-rate li a:hover” should be less than the z-index value of “.star-rate li a”). The link then has a background image load in with a background-position of 30px 60px so that only this part of the image will be displayed:
/*Same star image dimensions 30x30*/
width:30px;
height: 30px;
padding: 0px;
display:block;
/*Remove the underline from the link*/
text-decoration: none;
/*Indent the text off the screen (push the inner text off the screen)*/
text-indent: -8000px;
z-index: 10;
/* In the ul class “star-rate” we used relative position to allow us to use now the absolute position so that we can control the exact (x,y) coordinates of each star relative to the parent ul*/
position: absolute;
}
.star-rate li a:hover{
background: url(stars.gif) left;
/* Move this star to the bottom of the z-index stack */
z-index: 1;
/* Move this star all the way to the left */
left: 0px;
border:none;
background-position:30px 60px;
}

Each link on :hover should be as wide as its number of stars x 30px.
Example: for two stars we have: 2 x 30px = 60px, for three stars: 3 x 30px = 90px and so on.
This explains why we put a class on each different link.
CSS
.star-rate a.star-one{jQuery
left: 0px;
}
.star-rate a.star-one:hover{
width:30px;
}
.star-rate a.star-two{
left:30px;
}
.star-rate a.star-two:hover{
width: 60px;
}
.star-rate a.star-three{
left: 60px;
}
.star-rate a.star-three:hover{
width: 90px;
}
.star-rate a.star-four{
left: 90px;
}
.star-rate a.star-four:hover{
width: 120px;
}
.star-rate a.star-five{
left: 120px;
}
.star-rate a.star-five:hover{
width: 150px;
}
jQuery comes to our aid with the hover() command. When we hover over each link inside every list item we display its text inside the #remark id div and we remove the text as soon as we don’t hover over the link.
$(function(){
$( ".star-rate li a" ).each(function(i){
$(this).hover(function(){
$("#remark").append("<div id=\"core\">"+$(this).html()+"</p>");
},function(){
$("#core").remove();
});
});
});

Download Here
That’s it! Please feel free to comment

- Promote our sponsors;
- Add any kind of comment or critic;
- Ask me directly by email if you prefer.







If you tolerate an alternative HTML structure you can drop javascript requirement by using nested elements. See the code at http://paste.lisp.org/display/72647
It's not perfect, but it show the idea.
Tested with recent version of Firefox and Opera. Untested with IE. (I think it will not work with IE6.)
@ Anonymous,
I really like your HTML code (not working with IE7 as well). However, I am not sure that JS can be dropped as the point here is to use jQuery to ONLY show the every star’s description in the left of the page and nothing else.
Thanks a lot!